- How should I use torch.compile properly?
- How do I check if PyTorch is using the GPU?
- WARNING:tensorflow:Using a while_loop for converting cause there is no registered converter for this op
- How to use OneCycleLR?
- Error in Python script "Expected 2D array, got 1D array instead:"?
- How to save model in .pb format and then load it for inference in Tensorflow?
- Top 6 AI Logo Generator Up Until Now- Smarter Than Midjourney
- Best 9 AI Story Generator Tools
- The Top 6 AI Voice Generator Tools
- Best AI Low Code/No Code Tools for Rapid Application Development
- YOLOV8 how does it handle different image sizes
- Best AI Tools For Email Writing & Assistants
- 8 Data Science Competition Platforms Beyond Kaggle
- Data Analysis Books that You Can Buy
- Robotics Books that You Can Buy
- Data Visualization Books that You can Buy
- Digital image processing books that You Can Buy
- Natural Language Processing final year project ideas and guidelines
- OpenCV final year project ideas and guidelines
- Best Big Data Books that You Can Buy Today
How to set all tensors to cuda device?
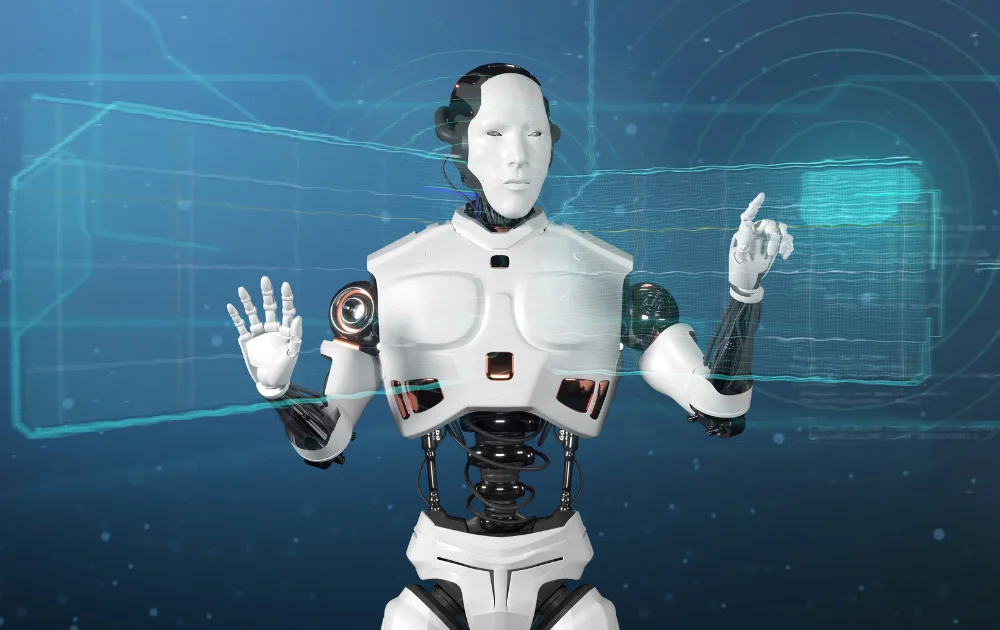
If you have GPU on our machine, then you can implement our model faster than CPU. But you have to set the tensors to the Cuda device so that the machine can utilize the GPU for training the model. Let's understand why we need to set tensors to the CUDA device and how to do it.
GPUs consist of thousands of small cores that perform optimization for parallel processing. Training deep learning models including matrix multiplication, and gradient computations becomes time-consuming on the CPU. That's why we need to move the tensors to the CUDA device so that the execution can be parallel and efficient. Modern GPUs give large amounts of high-speed memory. It is another advantage that helps in the fastest training.
There are several ways to set all the tensors to the CUDA device. We can use torch.cuda.set_device() function. Or we can use the .to() function to set all the tensors to the CUDA device.
first set the device name.
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
First example
import torch
torch.cuda.set_device(device)
# All tensors created after this line will be placed on the GPU by default
tensor = torch.randn(10, 10)
print(tensor.device)
Second example
import torch
tensor = torch.randn(10, 10)
# Move the tensor to the GPU
tensor = tensor.to(device)
print(tensor.device)
An Example can be how to set all the tensors to the CUDA device and train a Pytorch model on the CUDA device. Here, we define the device name. Then set tensor type to the device. Then create some data and set those to the device. Then perform neural network on those.
import torch
# Get the current CUDA device
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# Set the default tensor type to the CUDA device
torch.set_default_tensor_type(device)
# Create some data
x = torch.randn(10, 10)
y = torch.randn(10, 10)
# Move the data to the CUDA device
x = x.to(device)
y = y.to(device)
# Train the model
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
for epoch in range(10):
optimizer.zero_grad()
# Make a prediction
y_pred = model(x)
# Calculate the loss
loss = (y_pred - y).pow(2).mean()
# Backpropagate the loss
loss.backward()
# Update the model parameters
optimizer.step()
In the above code, first, you move the tensor into your device(CPU/CUDA), then perform model training with the specified data. If you have a GPU, then ensure that you have configured the CUDA and CuDNN accurately. Hope, you have gained precise knowledge about this topic.