- How to use OneCycleLR?
- Error in Python script "Expected 2D array, got 1D array instead:"?
- How to save model in .pb format and then load it for inference in Tensorflow?
- Top 6 AI Logo Generator Up Until Now- Smarter Than Midjourney
- Best 9 AI Story Generator Tools
- The Top 6 AI Voice Generator Tools
- Best AI Low Code/No Code Tools for Rapid Application Development
- YOLOV8 how does it handle different image sizes
- Best AI Tools For Email Writing & Assistants
- 8 Data Science Competition Platforms Beyond Kaggle
- Data Analysis Books that You Can Buy
- Robotics Books that You Can Buy
- Data Visualization Books that You can Buy
- Digital image processing books that You Can Buy
- Natural Language Processing final year project ideas and guidelines
- OpenCV final year project ideas and guidelines
- Best Big Data Books that You Can Buy Today
- Audio classification final year project ideas and guidelines
- How to print intercept and slope of a simple linear regression in Python with scikit-learn?
- How to take as Input a list of arrays in Keras API
WARNING:tensorflow:Using a while_loop for converting cause there is no registered converter for this op
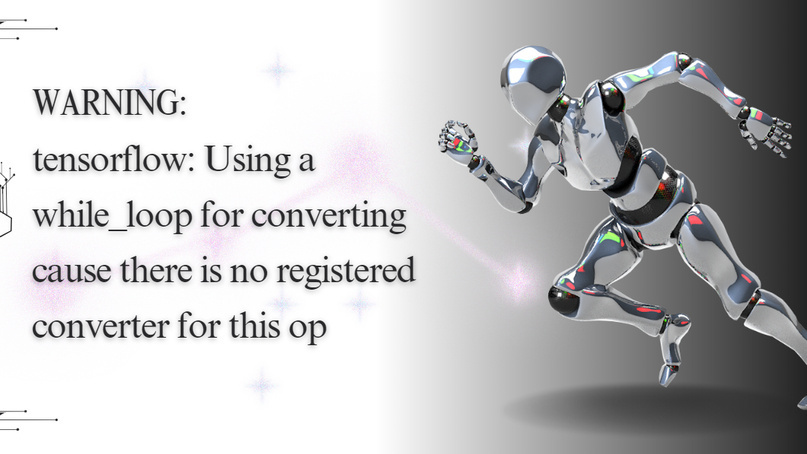
This type of error occurs due to the incompatible dependencies version of the device. There is a bug in Keras/TensorFlow 2.9 and 2.10, which causes preprocess layers like rescaling to be extremely slow. You can try the model without the rescaling layer. If you want to use this or similar preprocessing layers, you should use TF version 2.8.3 or older. It seems that some problem with the wrong version of TensorFlow/Keras/cuda.
Choose one stable version, and try again. Many times, the rescaling method occurs in error. If you want to use the rescaling method, then try this class instead of using the rescaling function from Keras.
class Rescaling(tf.keras.layers.Layer):
"""Multiply inputs by `scale` and adds `offset`. For instance: 1. To rescale an input in the `[0, 255]` range to be in the `[0, 1]` range,
you would pass `scale=1./255`. 2. To rescale an input in the `[0, 255]` range to be in the `[-1, 1]` range,
you would pass `scale=1./127.5, offset=-1`. The rescaling is applied both during training and inference.
Input shape:Arbitrary. Output shape:Same as input. Arguments: scale: Float, the scale to apply to the inputs. offset: Float, the offset to apply to the inputs. name: A string, the name of the layer. """
def __init__(self, scale, offset=0., name=None, **kwargs):
self.scale = scale
self.offset = offset
super(Rescaling, self).__init__(name=name, **kwargs)
def call(self, inputs):
dtype = self._compute_dtype
scale = tf.cast(self.scale, type)
offset = tf.cast(self.offset, type)
return tf.cast(inputs, dtype) * scale + offset
def compute_output_shape(self, input_shape):
return input_shape
def get_config(self):
config = { 'scale': self.scale, 'offset': self.offset, }
base_config = super(Rescaling, self).get_config()
return dict(list(base_config.items()) + list(config.items()))
You can also notice the preprocessing layers that are used for augmentation purposes. If the problem still remains, then ask the TensorFlow forum or the community. In summary, when you face issues with TensorFlow, Keras then be aware of potential bugs and compatibility concerns. Choose the right version of the libraries. If a Rescaling layer is used then use the custom "Rescaling" layer. Ensure a smoother and more efficient deep learning workflow.