- How to save model in .pb format and then load it for inference in Tensorflow?
- Top 6 AI Logo Generator Up Until Now- Smarter Than Midjourney
- Best 9 AI Story Generator Tools
- The Top 6 AI Voice Generator Tools
- Best AI Low Code/No Code Tools for Rapid Application Development
- YOLOV8 how does it handle different image sizes
- Best AI Tools For Email Writing & Assistants
- 8 Data Science Competition Platforms Beyond Kaggle
- Data Analysis Books that You Can Buy
- Robotics Books that You Can Buy
- Data Visualization Books that You can Buy
- Digital image processing books that You Can Buy
- Natural Language Processing final year project ideas and guidelines
- OpenCV final year project ideas and guidelines
- Best Big Data Books that You Can Buy Today
- Audio classification final year project ideas and guidelines
- How to print intercept and slope of a simple linear regression in Python with scikit-learn?
- How to take as Input a list of arrays in Keras API
- Tensorflow2.0 - How to convert Tensor to numpy() array
- How to train model for Background removal from images in Machine Learning
Error in Python script "Expected 2D array, got 1D array instead:"?
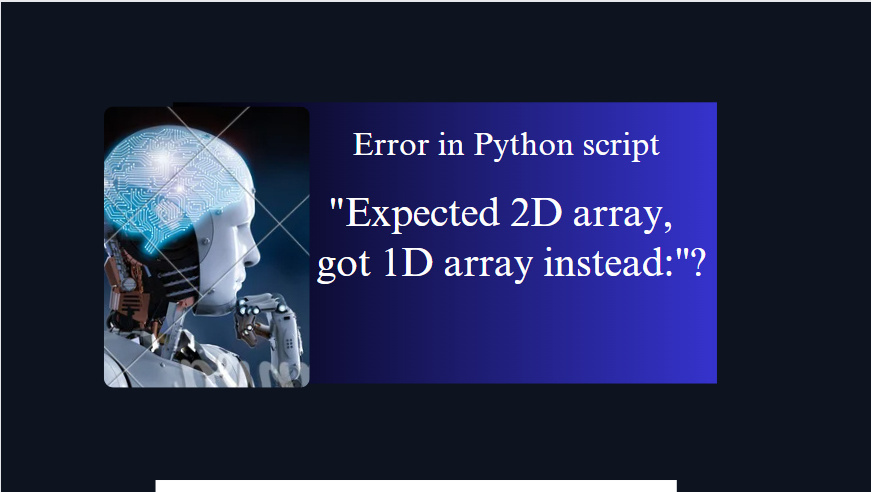
When working with arrays in Python, it's common to encounter errors that can disrupt your code execution. One such error many developers face is "ValueError: Expected 2D array, got 1D array instead." This error typically arises when you attempt to pass a 1D array to a function or method that expects a 2D array. This article will delve into the causes of this error and present multiple solutions to help you resolve it.
The "ValueError: Expected 2D array, got 1D array instead" error occurs when a function in your Python script expects a two-dimensional array but receives a one-dimensional array instead. In simpler terms, this error arises when you attempt to pass a 1D array into a function that requires a 2D array. This can be particularly challenging when working with libraries such as Scikit-learn in the context of machine learning.
Solution 1: Reshaping the Array
The first solution is to reshape the 1D array into the desired 2D array shape. Here's how you can do it:
Input
import numpy as np array_example_1d = np.array([1, 2, 3, 4, 5]) reshaped_array_result = np.reshape(array_example_1d, (5, 1)) print(reshaped_array_result)Output
[[1]
[2]
[3]
[4]
[5]]
By modifying the target shape to (5, 1), you obtain a 2D array with five rows and one column, resolving the value error.
Solution 2: Using the np.newaxis Attribute
Another solution is to use the np.newaxis attribute to convert a 1D array into a 2D array:
Input
import numpy as np
array_example_1d = np.array([1, 2, 3, 4, 5])
reshaped_array_output = array_example_1d[:, np.newaxis]
print(reshaped_array_output)
Solution 3: Using the np.expand_dims() Function
You can also use the np.expand_dims() function to convert a 1D array into a 2D array.
Input
import numpy as np
array_example_1d = np.array([10, 20, 30, 40, 50])
reshaped_array_output = np.expand_dims(array_example_1d, axis=1)
print(reshaped_array_output)
Solution:
The problem occurs when you run predictions on the array[0.58,0.76]
. Fix the problem by reshaping it before you call predict()
:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import style
style.use("ggplot")
from sklearn import svm
x = [1, 5, 1.5, 8, 1, 9]
y = [2, 8, 1.8, 8, 0.6, 11]
plt.scatter(x,y)
plt.show()
X = np.array([[1,2],
[5,8],
[1.5,1.8],
[8,8],
[1,0.6],
[9,11]])
y = [0,1,0,1,0,1]
clf = svm.SVC(kernel='linear', C = 1.0)
clf.fit(X,y)
test = np.array([0.58, 0.76])
print test # Produces: [ 0.58 0.76]
print test.shape # Produces: (2,) meaning 2 rows, 1 col
test = test.reshape(1, -1)
print test # Produces: [[ 0.58 0.76]]
print test.shape # Produces (1, 2) meaning 1 row, 2 cols
print(clf.predict(test)) # Produces [0], as expected
Output[0.58 0.76] (2,)
Although annoying, the Python "Expected 2D array, got 1D array instead" error is frequently encountered, mainly when using machine learning libraries like Scikit-learn. This error can be fixed, and script execution can be ensured by reshaping your data into a 2D array using techniques like wrapping it in double brackets or using the NumPy reshape function.
Python developers must comprehend and resolve this error since it has a direct bearing on how different Python libraries handle inputs and data. If you have the understanding to address this matter, you can make it more efficient and reduce the likelihood of mistakes in your Python scripting endeavor.
Thank you for reading this article.